mirror of
https://git.in.rschanz.org/ryan77627/guix.git
synced 2024-11-07 07:26:13 -05:00
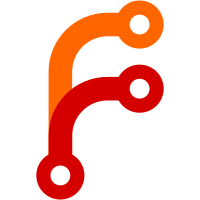
Less adding and more making it an actual bootloader rather than some weirdly specified packages. The GRUB EFI bootloader can be recreated by combining a Raspberry Pi bootloader with grub-efi. * gnu/bootloader.scm (efi-bootloader-profile, efi-bootloader-chain): Delete procedures. * gnu/bootloader/u-boot.scm (rpi-config, install-rpi, make-u-boot-rpi-bootloader): New procedures. (u-boot-rpi-2-bootloader, u-boot-rpi-3-bootloader, u-boot-rpi-4-bootloader, u-boot-rpi-bootloader): New variables. * gnu/packages/bootloaders.scm (make-u-boot-bin-package): Delete procedure. (%u-boot-rpi-efi-description, %u-boot-rpi-efi-description-32-bit, u-boot-rpi-2-efi, u-boot-rpi-3-32b-efi, u-boot-rpi-4-32b-efi, u-boot-rpi-arm64-efi, u-boot-rpi-2-bin, u-boot-rpi-3_32b-bin, u-boot-rpi-4_32b-bin, u-boot-rpi-arm64-bin, u-boot-rpi-2-efi-bin, u-boot-rpi-3-32b-efi-bin, u-boot-rpi-4-32b-efi-bin, u-boot-rpi-arm64-efi-bin): Delete variables. * gnu/packages/raspberry-pi.scm (grub-efi-bootloader-chain-raspi-64): Delete procedure. * gnu/system/examples/raspberry-pi-64-nfs-root.tmpl (bootloader), gnu/system/examples/raspberry-pi-64.tmpl (bootloader): Use new target system. Change-Id: I5139a0b00ec89189e8e7c84e06a7a3b7240259cd
303 lines
12 KiB
Scheme
303 lines
12 KiB
Scheme
;;; GNU Guix --- Functional package management for GNU
|
||
;;; Copyright © 2017 David Craven <david@craven.ch>
|
||
;;; Copyright © 2017, 2019 Mathieu Othacehe <m.othacehe@gmail.com>
|
||
;;; Copyright © 2020 Julien Lepiller <julien@lepiller.eu>
|
||
;;; Copyright © 2020 Jan (janneke) Nieuwenhuizen <janneke@gnu.org>
|
||
;;; Copyright © 2022 Maxim Cournoyer <maxim.cournoyer@gmail.com>
|
||
;;; Copyright © 2023 Efraim Flashner <efraim@flashner.co.il>
|
||
;;; Copyright © 2023-2024 Herman Rimm <herman@rimm.ee>
|
||
;;; Copyright © 2024 Zheng Junjie <873216071@qq.com>
|
||
;;; Copyright © 2024 Lilah Tascheter <lilah@lunabee.space>
|
||
;;;
|
||
;;; This file is part of GNU Guix.
|
||
;;;
|
||
;;; GNU Guix is free software; you can redistribute it and/or modify it
|
||
;;; under the terms of the GNU General Public License as published by
|
||
;;; the Free Software Foundation; either version 3 of the License, or (at
|
||
;;; your option) any later version.
|
||
;;;
|
||
;;; GNU Guix is distributed in the hope that it will be useful, but
|
||
;;; WITHOUT ANY WARRANTY; without even the implied warranty of
|
||
;;; MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
||
;;; GNU General Public License for more details.
|
||
;;;
|
||
;;; You should have received a copy of the GNU General Public License
|
||
;;; along with GNU Guix. If not, see <http://www.gnu.org/licenses/>.
|
||
|
||
(define-module (gnu bootloader u-boot)
|
||
#:use-module (gnu bootloader)
|
||
#:use-module (gnu bootloader extlinux)
|
||
#:use-module (gnu packages bootloaders)
|
||
#:use-module (gnu packages raspberry-pi)
|
||
#:use-module (gnu system boot)
|
||
#:use-module (guix gexp)
|
||
#:use-module (guix utils)
|
||
#:export (u-boot-a20-olinuxino-lime-bootloader
|
||
u-boot-a20-olinuxino-lime2-bootloader
|
||
u-boot-a20-olinuxino-micro-bootloader
|
||
u-boot-bananapi-m2-ultra-bootloader
|
||
u-boot-beaglebone-black-bootloader
|
||
u-boot-cubietruck-bootloader
|
||
u-boot-firefly-rk3399-bootloader
|
||
u-boot-mx6cuboxi-bootloader
|
||
u-boot-nanopi-r4s-rk3399-bootloader
|
||
u-boot-nintendo-nes-classic-edition-bootloader
|
||
u-boot-novena-bootloader
|
||
u-boot-orangepi-r1-plus-lts-rk3328-bootloader
|
||
u-boot-orangepi-zero2w-bootloader
|
||
u-boot-pine64-plus-bootloader
|
||
u-boot-pine64-lts-bootloader
|
||
u-boot-pinebook-bootloader
|
||
u-boot-pinebook-pro-rk3399-bootloader
|
||
u-boot-puma-rk3399-bootloader
|
||
u-boot-rock64-rk3328-bootloader
|
||
u-boot-rockpro64-rk3399-bootloader
|
||
u-boot-rpi-2-bootloader
|
||
u-boot-rpi-3-bootloader
|
||
u-boot-rpi-4-bootloader
|
||
u-boot-rpi-bootloader
|
||
u-boot-sifive-unmatched-bootloader
|
||
u-boot-qemu-riscv64-bootloader
|
||
u-boot-starfive-visionfive2-bootloader
|
||
u-boot-ts7970-q-2g-1000mhz-c-bootloader
|
||
u-boot-wandboard-bootloader))
|
||
|
||
(define (make-install-u-boot firmware installers)
|
||
(lambda* (#:key bootloader-config #:allow-other-keys . args)
|
||
(with-targets (bootloader-configuration-targets bootloader-config)
|
||
('extlinux (apply install-extlinux-config args))
|
||
(('install => (path :path)) #~(let ((path #$path)) #$firmware))
|
||
(('disk => (disk :device)) #~(let ((disk #$disk)) #f #$@installers)))))
|
||
|
||
(define-syntax-rule (define-u-bootloader def-name package firmware
|
||
(file size doffset) ...)
|
||
"Defines a U-Boot installer DEF-NAME, using u-boot PACKAGE. Installs
|
||
each given FILE of SIZE (or #f to autodetect) to the targeted disk at
|
||
OFFSET. FIRMWARE is ran on the U-Boot firmware directory to install
|
||
supporting files, with the directory path as the local variable 'path'."
|
||
(define def-name
|
||
(bootloader
|
||
(name 'u-boot)
|
||
(default-targets (list (bootloader-target
|
||
(type 'install)
|
||
(offset 'root)
|
||
(path "boot"))
|
||
(bootloader-target
|
||
(type 'extlinux)
|
||
(offset 'install)
|
||
(path "extlinux"))))
|
||
(installer
|
||
(make-install-u-boot
|
||
firmware
|
||
(list #~(let ((fw #$(file-append package "/libexec/" file)))
|
||
(write-file-on-device fw
|
||
#$(or size #~(stat:size (stat fw)))
|
||
disk #$doffset)) ...))))))
|
||
|
||
|
||
;;;
|
||
;;; Bootloader definitions.
|
||
;;;
|
||
|
||
(define-u-bootloader u-boot-beaglebone-black-bootloader
|
||
u-boot-am335x-boneblack #f
|
||
;; http://wiki.beyondlogic.org/index.php?title=BeagleBoneBlack_Upgrading_uBoot
|
||
;; This first stage bootloader called MLO (U-Boot SPL) is expected at
|
||
;; 0x20000 by BBB ROM code. The second stage bootloader will be loaded by
|
||
;; the MLO and is expected at 0x60000. Write both first stage ("MLO") and
|
||
;; second stage ("u-boot.img") images to the target.
|
||
("MLO" (* 256 512) (* 256 512))
|
||
("u-boot.img" (* 1024 512) (* 768 512)))
|
||
|
||
(define-u-bootloader u-boot-sifive-unmatched-bootloader
|
||
u-boot-sifive-unmatched #f
|
||
("spl/u-boot-spl.bin" #f (* 34 512))
|
||
("u-boot.itb" #f (* 2082 512)))
|
||
|
||
(define-u-bootloader u-boot-starfive-visionfive2-bootloader
|
||
u-boot-starfive-visionfive2
|
||
#~(begin (mkdir-p path)
|
||
(call-with-output-file (string-append path "/uEnv.txt")
|
||
(lambda (port)
|
||
(format port
|
||
;; if board SPI use vender's u-boot, will find
|
||
;; ""starfive/starfive_visionfive2.dtb"", We cannot guarantee
|
||
;; that users will update this u-boot, so set it.
|
||
"fdtfile=starfive/jh7110-starfive-visionfive-2-v1.3b.dtb~%"))))
|
||
("spl/u-boot-spl.bin.normal.out" #f (* 34 512))
|
||
("u-boot.itb" #f (* 2082 512)))
|
||
|
||
|
||
;;;
|
||
;;; Allwinner bootloader definitions.
|
||
;;;
|
||
(define-syntax-rule (define-u-bootloader-allwinner def-name package)
|
||
(define-u-bootloader def-name package #f
|
||
("u-boot-sunxi-with-spl.bin" #f (* 8 1024))))
|
||
|
||
|
||
(define-u-bootloader-allwinner u-boot-nintendo-nes-classic-edition-bootloader
|
||
u-boot-nintendo-nes-classic-edition)
|
||
|
||
(define-u-bootloader-allwinner u-boot-a20-olinuxino-lime-bootloader
|
||
u-boot-a20-olinuxino-lime)
|
||
|
||
(define-u-bootloader-allwinner u-boot-a20-olinuxino-lime2-bootloader
|
||
u-boot-a20-olinuxino-lime2)
|
||
|
||
(define-u-bootloader-allwinner u-boot-a20-olinuxino-micro-bootloader
|
||
u-boot-a20-olinuxino-micro)
|
||
|
||
(define-u-bootloader-allwinner u-boot-bananapi-m2-ultra-bootloader
|
||
u-boot-bananapi-m2-ultra)
|
||
|
||
(define-u-bootloader-allwinner u-boot-cubietruck-bootloader u-boot-cubietruck)
|
||
|
||
(define-u-bootloader-allwinner u-boot-pine64-lts-bootloader u-boot-pine64-lts)
|
||
|
||
(define-u-bootloader-allwinner u-boot-orangepi-zero2w-bootloader
|
||
u-boot-orangepi-zero2w)
|
||
|
||
|
||
;;;
|
||
;;; Allwinner64 bootloader definitions.
|
||
;;;
|
||
(define-syntax-rule (define-u-bootloader-allwinner64 def-name package)
|
||
(define-u-bootloader def-name package #f
|
||
("u-boot-sunxi-with-spl.bin" #f (* 8 1024))
|
||
("u-boot-sunxi-with-spl.fit.itb" #f (* 40 1024))))
|
||
|
||
(define-u-bootloader-allwinner64 u-boot-pine64-plus-bootloader
|
||
u-boot-pine64-plus)
|
||
|
||
(define-u-bootloader-allwinner64 u-boot-pinebook-bootloader u-boot-pinebook)
|
||
|
||
|
||
;;;
|
||
;;; IMX bootloader definitions.
|
||
;;;
|
||
(define-syntax-rule (define-u-bootloader-imx def-name package)
|
||
(define-u-bootloader def-name package #f
|
||
("SPL" #f (* 8 1024))
|
||
("u-boot.img" #f (* 40 1024))))
|
||
|
||
|
||
(define-u-bootloader-imx u-boot-mx6cuboxi-bootloader u-boot-mx6cuboxi)
|
||
|
||
(define-u-bootloader-imx u-boot-wandboard-bootloader u-boot-wandboard)
|
||
|
||
(define-u-bootloader-imx u-boot-novena-bootloader u-boot-novena)
|
||
|
||
|
||
;;;
|
||
;;; Rockchip bootloader definitions.
|
||
;;;
|
||
(define-syntax-rule (define-u-bootloader-rockchip def-name package)
|
||
;; SD and eMMC use the same format
|
||
(define-u-bootloader def-name package #f
|
||
("idbloader.img" #f (* 64 512))
|
||
("u-boot.itb" #f (* 16384 512))))
|
||
|
||
(define-u-bootloader-rockchip u-boot-firefly-rk3399-bootloader
|
||
u-boot-firefly-rk3399)
|
||
|
||
(define-u-bootloader-rockchip u-boot-nanopi-r4s-rk3399-bootloader
|
||
u-boot-nanopi-r4s-rk3399)
|
||
|
||
(define-u-bootloader-rockchip u-boot-orangepi-r1-plus-lts-rk3328-bootloader
|
||
u-boot-orangepi-r1-plus-lts-rk3328)
|
||
|
||
(define-u-bootloader-rockchip u-boot-rock64-rk3328-bootloader
|
||
u-boot-rock64-rk3328)
|
||
|
||
(define-u-bootloader-rockchip u-boot-rockpro64-rk3399-bootloader
|
||
u-boot-rockpro64-rk3399)
|
||
|
||
(define-u-bootloader-rockchip u-boot-pinebook-pro-rk3399-bootloader
|
||
u-boot-pinebook-pro-rk3399)
|
||
|
||
(define-u-bootloader u-boot-puma-rk3399-bootloader u-boot-puma-rk3399 #f
|
||
("idbloader.img" #f (* 64 512))
|
||
("u-boot.itb" #f (* 512 512)))
|
||
|
||
|
||
;;;
|
||
;;; Copy-only bootloader definitions.
|
||
;;;
|
||
|
||
;; These bootloaders don't really need to be installed, as they are read from
|
||
;; an SPI memory chip or directly from the FS, not the disk.
|
||
(define-syntax-rule (define-u-bootloader-copy def-name package file)
|
||
(define-u-bootloader def-name package
|
||
#~(install-file #$(file-append package "/libexec/" file) path)))
|
||
|
||
;; user should manually install this to SPI flash
|
||
;; TODO: write directly to SPI flash? unless wear issues are a problem.
|
||
(define-u-bootloader-copy u-boot-ts7970-q-2g-1000mhz-c-bootloader
|
||
u-boot-ts7970-q-2g-1000mhz-c "u-boot.imx")
|
||
|
||
(define-u-bootloader-copy u-boot-qemu-riscv64-bootloader
|
||
u-boot-qemu-riscv64 "u-boot.bin")
|
||
|
||
|
||
;;;
|
||
;;; Raspberry Pi bootloader definitions.
|
||
;;;
|
||
|
||
(define (rpi-config 64bit?)
|
||
"Raspberry Pi config.txt which includes a user-specified custom.txt."
|
||
(plain-file "config.txt"
|
||
(string-join
|
||
(list (string-append "arm_64bit=" (if 64bit? "1" "0"))
|
||
"enable_uart=1"
|
||
"kernel=u-boot.bin"
|
||
"include custom.txt")
|
||
#\newline
|
||
'suffix)))
|
||
|
||
(define (install-rpi u-boot-32 u-boot-64)
|
||
"Install the U-Boot from U-BOOT-64 for a 64-bit target, if available.
|
||
Otherwise install using U-BOOT-32."
|
||
(lambda* (#:key bootloader-config #:allow-other-keys . args)
|
||
(with-targets (bootloader-configuration-targets bootloader-config)
|
||
('install (apply install-extlinux-config args))
|
||
(('firmware => (firmware :path))
|
||
(let* ((32? (bootloader-configuration-32bit? bootloader-config))
|
||
(64bit? (and (not 32?) (target-64bit?) u-boot-64)))
|
||
#~(with-directory-excursion #$firmware
|
||
(atomic-copy #$(file-append (if 64bit? u-boot-64 u-boot-32)
|
||
"/libexec/u-boot.bin")
|
||
"u-boot.bin")
|
||
(atomic-copy #$(rpi-config 64bit?) "config.txt")))))))
|
||
|
||
(define* (make-u-boot-rpi-bootloader #:key u-boot-32 u-boot-64)
|
||
"Make a Raspberry Pi bootloader using either U-BOOT-32 or U-BOOT-64."
|
||
(bootloader (name 'u-boot)
|
||
(default-targets
|
||
(list (bootloader-target (type 'install)
|
||
(offset 'firmware)
|
||
(path "extlinux"))
|
||
(bootloader-target (type 'firmware)
|
||
(offset 'root)
|
||
(path "boot"))))
|
||
(installer (install-rpi u-boot-32 u-boot-64))))
|
||
|
||
;; These neither install firmware nor device-tree files for the Raspberry Pi.
|
||
;; They just assume them to be existing in 'install in the same way that some
|
||
;; UEFI firmware with ACPI data is usually assumed to be existing on PCs.
|
||
;; They can be used with either extlinux or as UEFI firmware, alongside
|
||
;; e.g. GRUB.
|
||
(define u-boot-rpi-2-bootloader
|
||
(make-u-boot-rpi-bootloader #:u-boot-32 u-boot-rpi-2))
|
||
|
||
(define u-boot-rpi-3-bootloader
|
||
(make-u-boot-rpi-bootloader #:u-boot-32 u-boot-rpi-3-32b
|
||
#:u-boot-64 u-boot-rpi-arm64))
|
||
|
||
(define u-boot-rpi-4-bootloader
|
||
(make-u-boot-rpi-bootloader #:u-boot-32 u-boot-rpi-4-32b
|
||
#:u-boot-64 u-boot-rpi-arm64))
|
||
|
||
;; Usable for any 64-bit Raspberry Pi.
|
||
(define u-boot-rpi-bootloader
|
||
(make-u-boot-rpi-bootloader #:u-boot-64 u-boot-rpi-arm64))
|